Rainbow Face
Face color depends from the current time
Description
Face color of this clock depends from the current time. Simplest code fills the face by the new color using OnBeforeDrawFaceListener of TrueAnalogClock class.
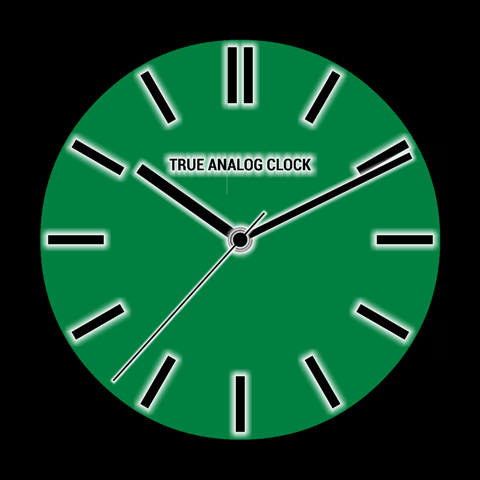
Anatomy
Transparent face and simple standard hands. Hands and indices of the face have white shadows.
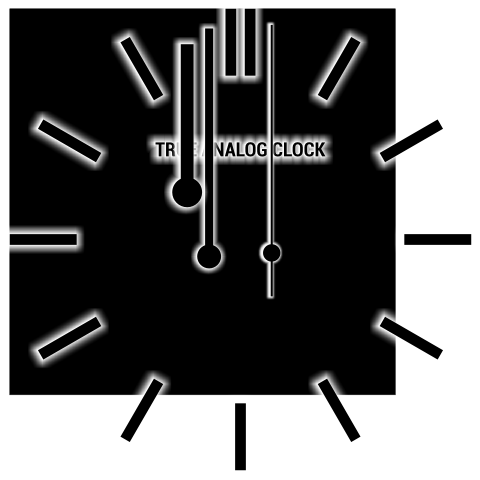
XML Settings File
<?xml version="1.0" encoding="utf-8"?>
<TrueAnalogClock
face="rainbow_face_face"
useSystemTime="true">
<hand
image="rainbow_face_hand_hour"
axisFacePoint="1075:1075"
axisPoint="101:697"
type="Hour"/>
<hand
image="rainbow_face_hand_minute"
axisFacePoint="1075:1075"
axisPoint="81:1049"
type="Minute"/>
<hand
image="rainbow_face_hand_second"
axisFacePoint="1075:1075"
axisPoint="59:1039"
type="Second"/>
</TrueAnalogClock>
Manual code
The code sets listener and draw the colored circle before drawing the face image.
TrueAnalogClock.BeforeDrawFaceListener listener =
new TrueAnalogClock.BeforeDrawFaceListener() {
@Override
public Boolean onBeforeDrawFace(TrueAnalogClock clock, Canvas canvas) {
calendar.setTimeInMillis(clock.getTime());
float[] hsv = new float[3];
hsv[0] = 360 / 24 * calendar.get(Calendar.HOUR_OF_DAY);
hsv[1] = 1;
hsv[2] = 0.5f;
Paint p = new Paint();
p.setColor(Color.HSVToColor(hsv));
p.setStyle(Paint.Style.FILL);
canvas.drawCircle(canvas.getWidth() / 2, canvas.getHeight() / 2,
canvas.getWidth() / 2, p);
return false;
}
};
clock.setBeforeDrawFaceListener(listener);